Local Storage seems to be disabled in your browser.
For the best experience on our site, be sure to turn on Local Storage in your browser.
A Note About 'X-Magento-Tags'
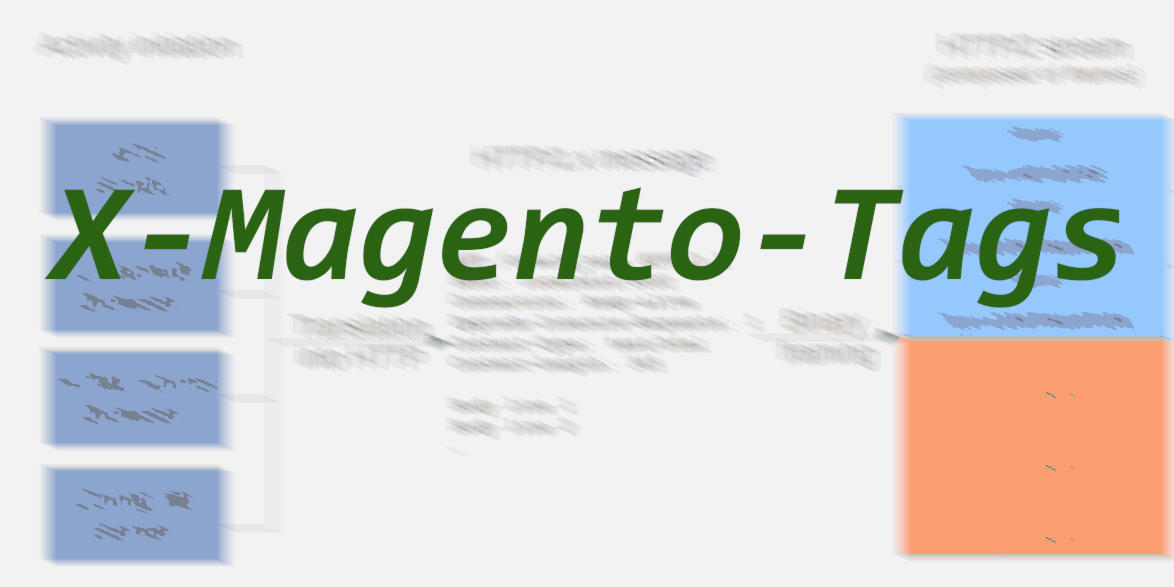
If you debugged Magento, I believe you have ever seen the 'X-Magento-Tags' in the response headers. What is 'X-Magento-Tags' used for? How is the 'X-Magento-Tags' generated?
We will cover these questions in this article and in fact 'X-Magento-Tags' header is also used in ESI block responses. They are the keys when you purge Varnish or Fastly.
The core part:
<?php
# File: Magento\PageCache\Model\Layout\LayoutPlugin
public function afterGetOutput(Layout $subject, $result)
{
if ($subject->isCacheable() && $this->config->isEnabled()) {
$tags = [];
$isVarnish = $this->config->getType() === Config::VARNISH;
foreach ($subject->getAllBlocks() as $block) {
if ($block instanceof IdentityInterface) {
$isEsiBlock = $block->getTtl() > 0;
if ($isVarnish && $isEsiBlock) {
continue;
}
$tags[] = $block->getIdentities();
}
}
$tags = array_unique(array_merge([], ...$tags));
$tags = $this->pageCacheTagsPreprocessor->process($tags);
$this->response->setHeader('X-Magento-Tags', implode(',', $tags));
}
return $result;
}
As you can see, $tags[] = $block->getIdentities();, tags come from "identities" of block.
So to let your block have tags, you need to let your block implement \Magento\Framework\DataObject\IdentityInterface and create your getIdentities() method. There are many examples in Magento core modules, eg: File: Magento\Cms\Block\Page
But you should notice that if full page cache is handled by Varnish or block is an ESI block, then those tags should not be included in response. WHY???
To answer this question, first you need to know what is ESI and how it works.
The ESI stands for Edge Side Includes. The cached main page HTML contains ESI blocks and they will be replaced by Varnish when Varnish sends the final response. You may wonder why programmers made this design. The benefit is that the ESI block can have a different lifetime with the main page.
And you should notice that the ESI block has 'X-Magento-Tags'.
See the code below:
<?php
# File: Magento\PageCache\Controller\Block\Esi
public function execute()
{
$response = $this->getResponse();
$blocks = $this->_getBlocks();
$html = '';
$ttl = 0;
if (!empty($blocks)) {
$blockInstance = array_shift($blocks);
$html = $blockInstance->toHtml();
$ttl = $blockInstance->getTtl();
if ($blockInstance instanceof \Magento\Framework\DataObject\IdentityInterface) {
$response->setHeader('X-Magento-Tags', implode(',', $blockInstance->getIdentities()));
}
}
$this->translateInline->processResponseBody($html);
$response->appendBody($html);
$response->setPublicHeaders($ttl);
}
So you can purge just a block rather than a whole page content.
The most typical usage is the navigation bar. When you edit categories, only the navigation bar block will be purged, otherwise every page should be purged as the navigation bar almost appears on every page.
OK, we learned one more point on how to gain your store's performance, we hope you can understand the Magento caching system better and how it cooperates with Varnish(and Fastly).