Local Storage seems to be disabled in your browser.
For the best experience on our site, be sure to turn on Local Storage in your browser.
How does Full Page Cache(FPC) work?
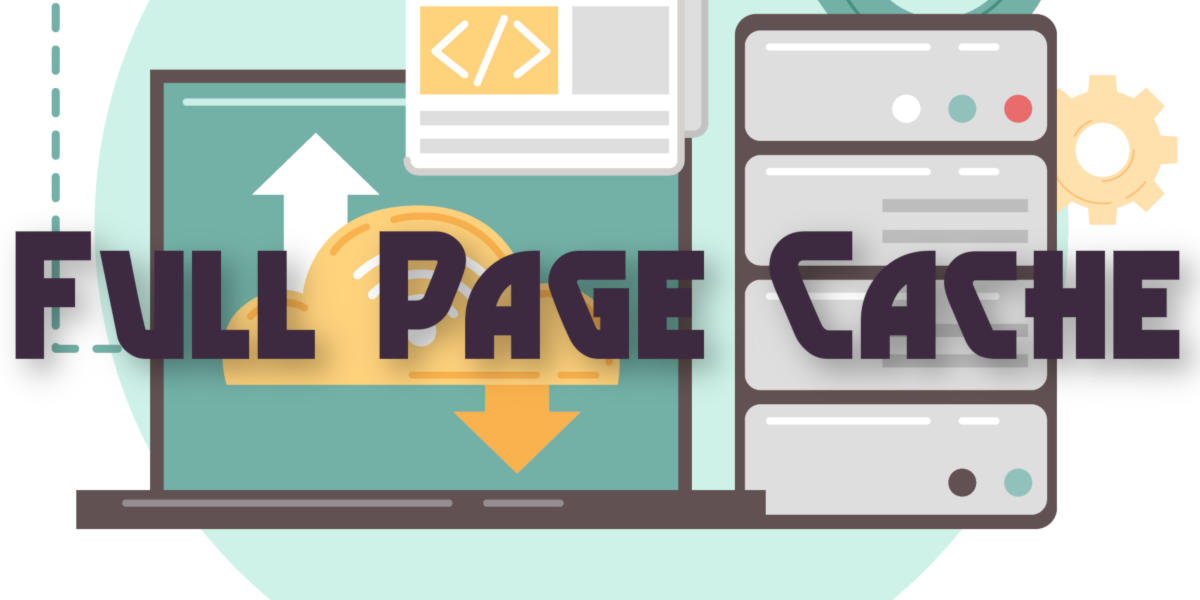
Full Page Cache is one core feature that significantly boost the speed of your store. It comes default with Magento 2. When the Cache is hit, Magento can skip FrontController and return the response. So how does this work in code level?
The core part is anew around plugin for FrontController.
<?php
# File: Magento\PageCache\Model\App\FrontController\BuiltinPlugin
public function aroundDispatch(
\Magento\Framework\App\FrontControllerInterface $subject,
\Closure $proceed,
\Magento\Framework\App\RequestInterface $request
) {
$this->version->process();
if (!$this->config->isEnabled() || $this->config->getType() !== \Magento\PageCache\Model\Config::BUILT_IN) {
return $proceed($request);
}
$result = $this->kernel->load();
if ($result === false) {
$result = $proceed($request);
if ($result instanceof ResponseHttp && !$result instanceof NotCacheableInterface) {
$this->addDebugHeaders($result);
$this->kernel->process($result);
}
} else {
$this->addDebugHeader($result, 'X-Magento-Cache-Debug', 'HIT', true);
}
return $result;
}
$result = $this->kernel->load(); loads the page cache.
If $result is not false, that is, the page cache was found, this plugin won't call $proceed, which means the process in FrontController is skipped. This part shows how to use cache to increase performance.
$this->addDebugHeader($result, 'X-Magento-Cache-Debug', 'HIT', true); Add debug header in developer mode.
$result = $this->kernel->process(); saves the page cache.
The conditions for saving page cache are:
HEAD or GET request
The response is HTML Page
The response HTTP status code is 200
If you go deeper by looking at Magento\PageCache\Model\App\PageCache\Kernel and Magento\PageCache\Model\App\PageCache\Identifier
<?php
# File: Magento\PageCache\Model\App\PageCache\Kernel
public function process(\Magento\Framework\App\Response\Http $response)
{
$cacheControlHeader = $response->getHeader('Cache-Control');
if ($cacheControlHeader
&& preg_match('/public.*s-maxage=(\d+)/', $cacheControlHeader->getFieldValue(), $matches)
) {
$maxAge = $matches[1];
$response->setNoCacheHeaders();
if (($response->getHttpResponseCode() == 200 || $response->getHttpResponseCode() == 404)
&& !$response instanceof NotCacheableInterface
&& ($this->request->isGet() || $this->request->isHead())
) {
$tagsHeader = $response->getHeader('X-Magento-Tags');
$tags = $tagsHeader ? explode(',', $tagsHeader->getFieldValue() ?? '') : [];
$response->clearHeader('Set-Cookie');
if ($this->state->getMode() != AppState::MODE_DEVELOPER) {
$response->clearHeader('X-Magento-Tags');
}
$this->cookieDisabler->setCookiesDisabled(true);
$this->fullPageCache->save(
$this->serializer->serialize($this->getPreparedData($response)),
$this->identifierForSave->getValue(),
$tags,
$maxAge
);
}
}
}
<?php
# File: Magento\PageCache\Model\App\PageCache\Identifier
public function getValue()
{
$data = [
$this->request->isSecure(),
$this->request->getUriString(),
$this->request->get(\Magento\Framework\App\Response\Http::COOKIE_VARY_STRING)
?: $this->context->getVaryString()
];
return sha1($this->serializer->serialize($data));
}
You can also notice that the page cache depends on the 'X-Magento-Vary' cookie by examine $this->request->get(\Magento\Framework\App\Response\Http::COOKIE_VARY_STRING) ?: $this->context->getVaryString().
In this article, we have introduced the core part of Magento Full Page Cache.
However, to maximize your store's performance, we recommend you to have a Varnish server or even employ CDN.