Local Storage seems to be disabled in your browser.
For the best experience on our site, be sure to turn on Local Storage in your browser.
How to display a notice when customer selects certain Payment Method in Magento 2?
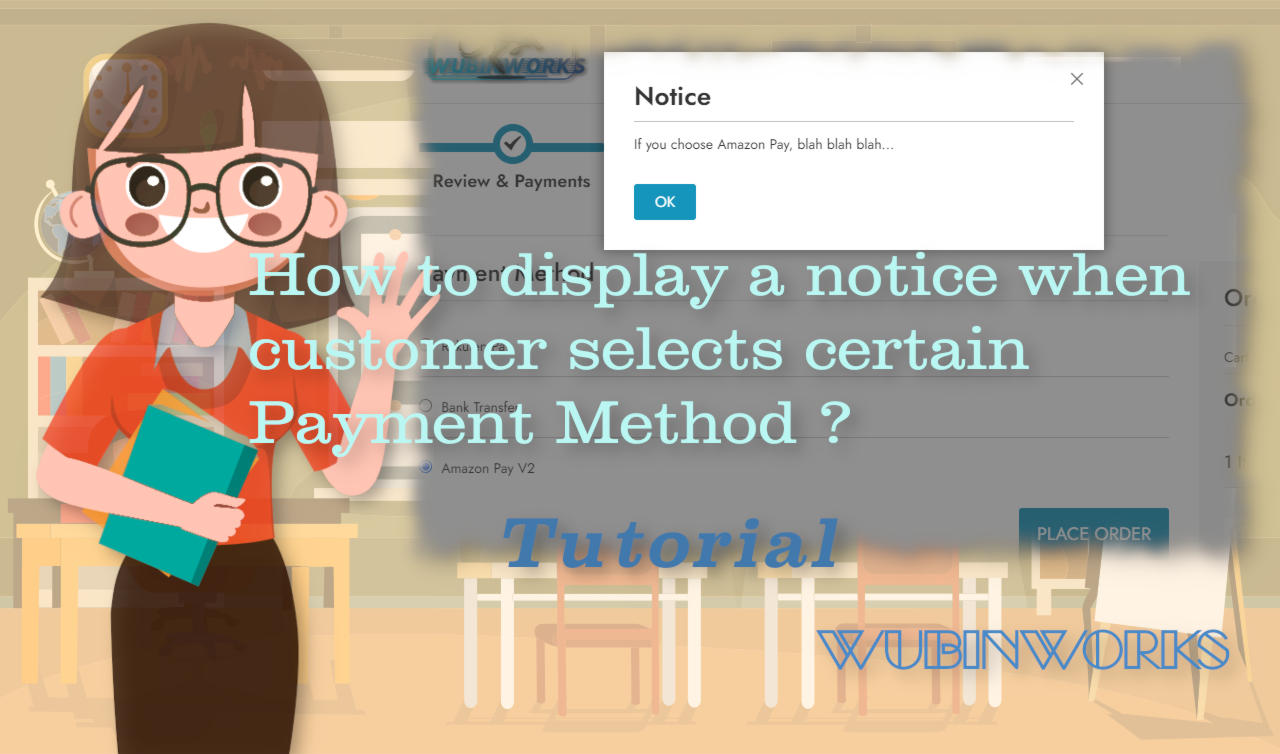
Magento supports multiple payment methods and you can even develop your own payment method. This is a big plus as store owners can fully control the last and the most important step of checkout process. However, in many cases, we just need a simple customization for existing payment methods. One common request is to display a notice when customer selects a certain payment method. The purpose varies from store to store. Some stores may notify the customer this payment method overrides the default return policy. Some stores may inform that it will take extra 1 ~ 2 days to verify the payment before shipping. Now let's focus on how to do it in "Magento Way".
Prerequisites
- Know how to initiate a javascript in Magento
- Be familiar with knockout.js
Implementation
Though this is a tutorial module, we are going to make it as flexible as possible so that it may have some practical use.
We use Amazon Pay as an example and the code was tested on Magento 2.4.6 and Magento 2.4.7.
When customer selects Amazon Pay, or the Amazon Pay is pre-selected, a dialog(popup) will be displayed. See the blog post image to find out what it will look like.
Step 1
Find the payment method code of Amazon Pay.
We found it in the etc/payment.xml and the code is amazon_payment_v2
.
Step 2
Open a new Magento module Wubinworks_Tutorial
.
Inject a block to layout in checkout_index_index
action. This block is just for initiating a javascript.
<?xml version="1.0"?>
<!--
/**
* Copyright © Wubinworks. All rights reserved.
*
* NOTICE OF LICENSE
*
* This source file is subject to the Open Software License (OSL-3.0)
* that is available through the world-wide-web at this URL:
* https://opensource.org/licenses/OSL-3.0
*
* @author Wubinworks Dev Team
* @copyright Wubinworks
* @license https://opensource.org/licenses/OSL-3.0 Open Software License (OSL-3.0)
* @link https://www.wubinworks.com/contact Contact us
*/
-->
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceBlock name="content">
<block class="Magento\Framework\View\Element\Template"
name="wubinworks_tutorial_checkout_payment_method_notice"
template="Wubinworks_Tutorial::checkout/payment-method-notice.phtml"
/>
</referenceBlock>
</body>
</page>
Step 3
Initiate the javascript with configuration.
<?php
/**
* Copyright © Wubinworks. All rights reserved.
*
* NOTICE OF LICENSE
*
* This source file is subject to the Open Software License (OSL-3.0)
* that is available through the world-wide-web at this URL:
* https://opensource.org/licenses/OSL-3.0
*
* @author Wubinworks Dev Team
* @copyright Wubinworks
* @license https://opensource.org/licenses/OSL-3.0 Open Software License (OSL-3.0)
* @link https://www.wubinworks.com/contact Contact us
*/
?>
<?php
// Your dialog configuration
$config = json_encode([
'code' => 'amazon_payment_v2',
'title' => __('Notice'),
'message' => __('If you choose Amazon Pay, blah blah blah...')
], JSON_HEX_TAG);
?>
<script type="text/x-magento-init">
{
"*": {
"Wubinworks_Tutorial/js/checkout/payment-method-notice": <?= /** @noEscape */ $config ?>
}
}
</script>
As you can check the quote model has a paymentMethod
observable and it is changed here, our customization is based on these locations.
/**
* Copyright © Wubinworks. All rights reserved.
*
* NOTICE OF LICENSE
*
* This source file is subject to the Open Software License (OSL-3.0)
* that is available through the world-wide-web at this URL:
* https://opensource.org/licenses/OSL-3.0
*
* @author Wubinworks Dev Team
* @copyright Wubinworks
* @license https://opensource.org/licenses/OSL-3.0 Open Software License (OSL-3.0)
* @link https://www.wubinworks.com/contact Contact us
*/
define([
'ko',
'Magento_Checkout/js/model/quote',
'Magento_Ui/js/modal/alert'
], function (ko, Quote, Alert) {
'use strict';
return function (config) {
/**
* Execute
* @private
*/
function execute() {
Quote.paymentMethod.subscribe(function (newValue) {
// You may record whether the dialog has been showed before in Local Storage
if (newValue && newValue.method === config.code) {
Alert({
title: config.title,
content: config.message
});
}
}, null, 'change');
}
execute();
};
});
The key point is to use observable's subscribe method to "detect" payment method change.
Now you can test and adjust this module.
Final Thoughts
This module only do extreme basic things, you may want to only display the notice for once or employ other logics, but anyway, you can use it as a starting point.
If this tutorial did help you, please share this blog post.