Local Storage seems to be disabled in your browser.
For the best experience on our site, be sure to turn on Local Storage in your browser.
How to get config data directly from `config.xml` in Magento 2
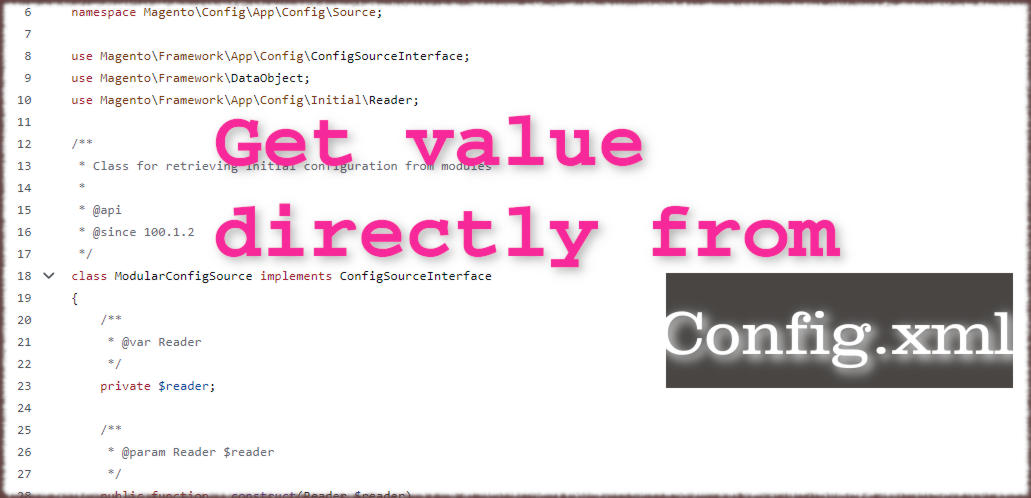
Magento 2 uses "Scoped configuration" to adopt multiple stores setup. Configuration value has a fallback mechanism and the "default value" is stored in etc/config.xml
. In most cases, we just make use of that fallback mechanism as we want the value for a specific store. But what if we want to retrieve the value directly from config.xml
? In short, the answer is ModularConfigSource
. Let's see how to achieve it.
Traditional Way
Get a user specified value and check the value. If the value is not appropriate, use a hardcoded value instead.
public function getSomeConfigValue()
{
...
/** @var \Magento\Framework\App\Config\ScopeConfigInterface $scopeConfig */
$value = $scopeConfig->getValue('section1/group1/field1');
if (!check_value($value)) {
return 'hardcoded_value';
}
return $value;
}
You also need to keep the hardcoded_value
the same in config.xml
.
But in Some Situations...
In some cases, Magento cannot validate the user specified value when saving the configuration, the value can only be checked at runtime.
New Way
Inject dependency \Magento\Config\App\Config\Source\ModularConfigSource
.
public function getSomeConfigValue()
{
...
/** @var \Magento\Framework\App\Config\ScopeConfigInterface $scopeConfig */
$value = $scopeConfig->getValue('section1/group1/field1');
if (!check_value_at_runtime($value)) {
/** @var \Magento\Config\App\Config\Source\ModularConfigSource $modularConfigSource */
return $modularConfigSource->get('default/' . 'section1/group1/field1');
}
return $value;
}
$modularConfigSource->get('default/' . 'section1/group1/field1')
returns the value directly from config.xml
, no fallback mechanism. So you can store an absolutely working value in config.xml
.
Don't forget to add the 'default/'
prefix.
Advantage of New Way
There are 2 main advantages:
-
If another developer wants to override your module, it is much easier to override the
config.xml
rather thanpreference
the PHP class. -
Just one place to maintain the "default absolutely working value".
We hope this blog post can help someone's development.